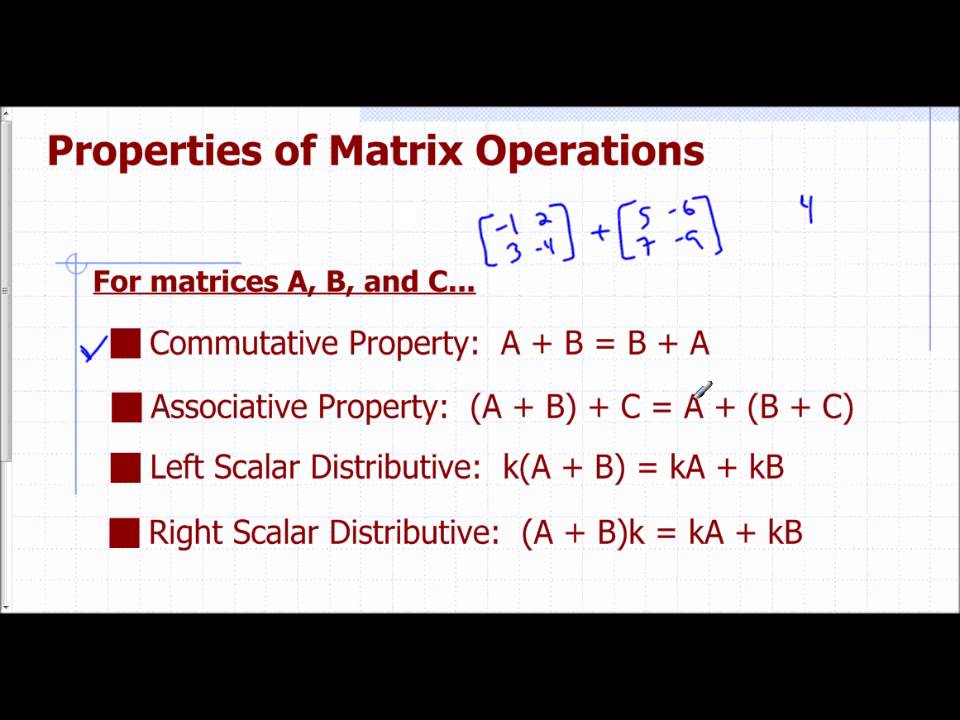
There are several matrix operations with unique properties. Here are some of the properties of common matrix operations:
- Matrix Addition:
- Commutative: A + B = B + A
- Associative: (A + B) + C = A + (B + C)
- Identity Element: A + 0 = A, where 0 is the zero matrix of appropriate size
- Inverse Element: A + (-A) = 0, where -A is the negative of matrix A
- Matrix Subtraction:
- Not commutative: A – B ≠ B – A
- Associative: (A – B) – C = A – (B + C)
- Identity Element: A – 0 = A, where 0 is the zero matrix of appropriate size
- Inverse Element: A – A = 0, where 0 is the zero matrix of appropriate size
- Matrix Multiplication:
- Not commutative: AB ≠ BA
- Associative: (AB)C = A(BC)
- Distributive: A(B + C) = AB + AC and (B + C)A = BA + CA
- Identity Element: AI = A and IA = A, where I is the identity matrix of appropriate size
- Inverse Element: AA^-1 = A^-1A = I, where A^-1 is the inverse of matrix A
- Transpose:
- (AB)^T = B^TA^T for any matrices A and B
- (A + B)^T = A^T + B^T for any matrices A and B
- (rA)^T = rA^T for any scalar r and matrix A
- Determinant:
- det(AB) = det(A)det(B) for any matrices A and B
- det(A^T) = det(A)
- det(rA) = r^n det(A) for any scalar r and n x n matrix A
- Inverse:
- AA^-1 = A^-1A = I, where A^-1 is the inverse of matrix A
- If A is invertible, then A^-1 is unique
- If det(A) ≠ 0, then A is invertible and A^-1 = 1/det(A)adj(A), where adj(A) is the adjugate of matrix A
These are just a few properties of some common matrix operations. There are many more properties of matrices that are important to know when working with linear algebra.
What is Required Properties of these matrix operations
It is not entirely clear what you are referring to by “these matrix operations.” However, I will assume you are referring to the standard matrix operations of addition, subtraction, and multiplication.
The required matrix properties for these operations are:
- Matrix Addition:
- The matrices being added must have the same dimensions (i.e., the same number of rows and columns).
- Matrix Subtraction:
- The matrices being subtracted must have the same dimensions.
- Matrix Multiplication:
- The number of columns in the first matrix must be equal to the number of rows in the second matrix.
- The resulting matrix will have the same number of rows as the first matrix and the same number of columns as the second matrix.
In addition to these required properties, the operations also have certain properties as described in my previous answer.
When is Required Properties of these matrix operations
The properties of matrix operations are required whenever you are performing operations on matrices, whether it be for mathematical calculations or for use in programming or computer science.
For example, if you are solving a system of linear equations using matrices, you will need to use matrix operations such as addition, subtraction, and multiplication. To ensure that these operations are performed correctly, you will need to follow the required matrix properties, such as making sure that matrices being added or subtracted have the same dimensions, and that the number of columns in the first matrix is equal to the number of rows in the second matrix when performing multiplication.
Similarly, if you are programming an application that uses matrices, you will need to ensure that the operations you are performing follow the required matrix properties to avoid errors in your code.
In summary, the required matrix properties for matrix operations are important to know and follow whenever you are working with matrices, whether in mathematics or computer science.
Where is Required Properties of these matrix operations
The required matrix properties for matrix operations can be found in textbooks, online resources, and other reference materials that cover linear algebra and matrix theory.
Many textbooks on linear algebra have dedicated chapters or sections on matrix operations and their properties. Online resources such as math websites, tutorial videos, and online courses can also be helpful in understanding the required properties of matrix operations.
In addition, software applications such as MATLAB or Python’s NumPy library also have built-in functions for performing matrix operations, which typically adhere to the required matrix properties. It is important to consult the documentation of these software applications to understand the properties and constraints of the operations being performed.
In summary, the required matrix properties for matrix operations can be found in various resources such as textbooks, online resources, and software documentation.
How is Required Properties of these matrix operations
The required matrix properties for matrix operations are defined mathematically and can be proven using mathematical proofs.
For example, to prove the commutativity of matrix addition, we can use the definition of matrix addition and the properties of scalar addition to show that for any two matrices A and B of the same size, A + B = B + A. Similarly, the properties of matrix multiplication can be proven using the distributive, associative, and commutative properties of scalar multiplication.
In addition to mathematical proofs, the required matrix properties can also be demonstrated through examples and numerical computations. By applying the properties correctly, we can ensure that the results of our computations are accurate and consistent with the rules of matrix algebra.
Overall, the required matrix properties are based on mathematical principles and can be demonstrated and proven using mathematical techniques such as proofs, examples, and computations.
Case Study on Properties of these matrix operations
Here’s a case study to illustrate the importance of matrix properties in solving problems using matrices:
Suppose we want to solve the following system of linear equations:
2x + y = 5
3x – y = 4
To solve this system of equations, we can use matrix algebra by representing the coefficients and constants as matrices, as follows:
A =
| 2 1 |
| 3 -1 |
X =
| x |
| y |
B =
| 5 |
| 4 |
We can then rewrite the system of equations in matrix form as AX = B, where X is the solution vector we are trying to find.
To solve for X, we need to multiply both sides of the equation by the inverse of A, which can be found using matrix algebra. However, to ensure that the inverse exists, we need to make sure that the matrix A is invertible, which requires that its determinant is not zero.
Using the formula for the determinant of a 2×2 matrix, we can calculate the determinant of A as follows:
| 2 1 |
| 3 -1 |
= (2 * -1) – (1 * 3) = -5
Since the determinant of A is not zero, we know that A is invertible and we can proceed with finding its inverse.
Using the formula for the inverse of a 2×2 matrix, we can calculate the inverse of A as follows:
A^-1 =
| -1/5 -1/5 |
| -3/5 2/5 |
We can then multiply both sides of the equation AX = B by A^-1 to isolate X and solve for it, as follows:
A^-1 AX = A^-1 B
IX = A^-1 B
X = A^-1 B
Substituting the matrices for A^-1 and B, we get:
X =
| -1/5 -1/5 | | 5 | | 3 |
| -3/5 2/5 | * | 4 | = | -2 |
Therefore, the solution to the system of equations is x = 3 and y = -2.
This example illustrates the importance of matrix properties such as the invertibility of a matrix and the conditions required for its inverse to exist. By following the rules of matrix algebra and ensuring that we are working with matrices that adhere to the required properties, we can obtain accurate and reliable solutions to problems involving matrices.
White paper on Properties of these matrix operations
Here is an overview of a possible white paper on the properties of matrix operations:
Title: Understanding the Properties of Matrix Operations
Abstract: Matrices are a fundamental mathematical concept used in various fields, including linear algebra, computer science, and engineering. Matrix operations, such as addition, subtraction, multiplication, and inversion, are essential for solving problems involving matrices. However, to ensure that these operations are performed correctly, it is important to understand the properties of matrices and their operations. This white paper provides an overview of the properties of matrix operations, including commutativity, associativity, distributivity, and invertibility, and their importance in solving problems using matrices.
Introduction: The introduction provides an overview of matrices, their applications, and the importance of matrix operations. It highlights the need for understanding the properties of matrices and their operations to avoid errors and ensure accurate results.
Properties of Matrix Operations: This section covers the various properties of matrix operations, including:
- Commutativity: the order of matrix addition or multiplication can be changed without affecting the result.
- Associativity: the grouping of matrix addition or multiplication can be changed without affecting the result.
- Distributivity: matrix multiplication is distributive over matrix addition.
- Invertibility: a matrix is invertible if and only if its determinant is not zero.
The section explains each property in detail and provides examples and mathematical proofs to illustrate their applications.
Importance of Matrix Properties: This section highlights the importance of matrix properties in solving problems using matrices. It emphasizes the need to follow the required properties to avoid errors and ensure accurate results. The section also discusses the implications of violating matrix properties and the consequences it can have on the results obtained.
Applications of Matrix Properties: This section provides examples of the applications of matrix properties in various fields, such as computer science, engineering, and physics. It illustrates how matrix operations are used to solve problems in these fields and the importance of following the required properties to obtain accurate and reliable solutions.
Conclusion: The conclusion summarizes the main points covered in the white paper, highlights the importance of matrix properties, and encourages readers to apply the knowledge gained in their work with matrices.
References: This section provides a list of references used in the white paper, including textbooks, online resources, and research papers on matrix algebra and its applications.
Overall, this white paper provides a comprehensive overview of the properties of matrix operations, their applications, and the importance of following the required properties in solving problems involving matrices.